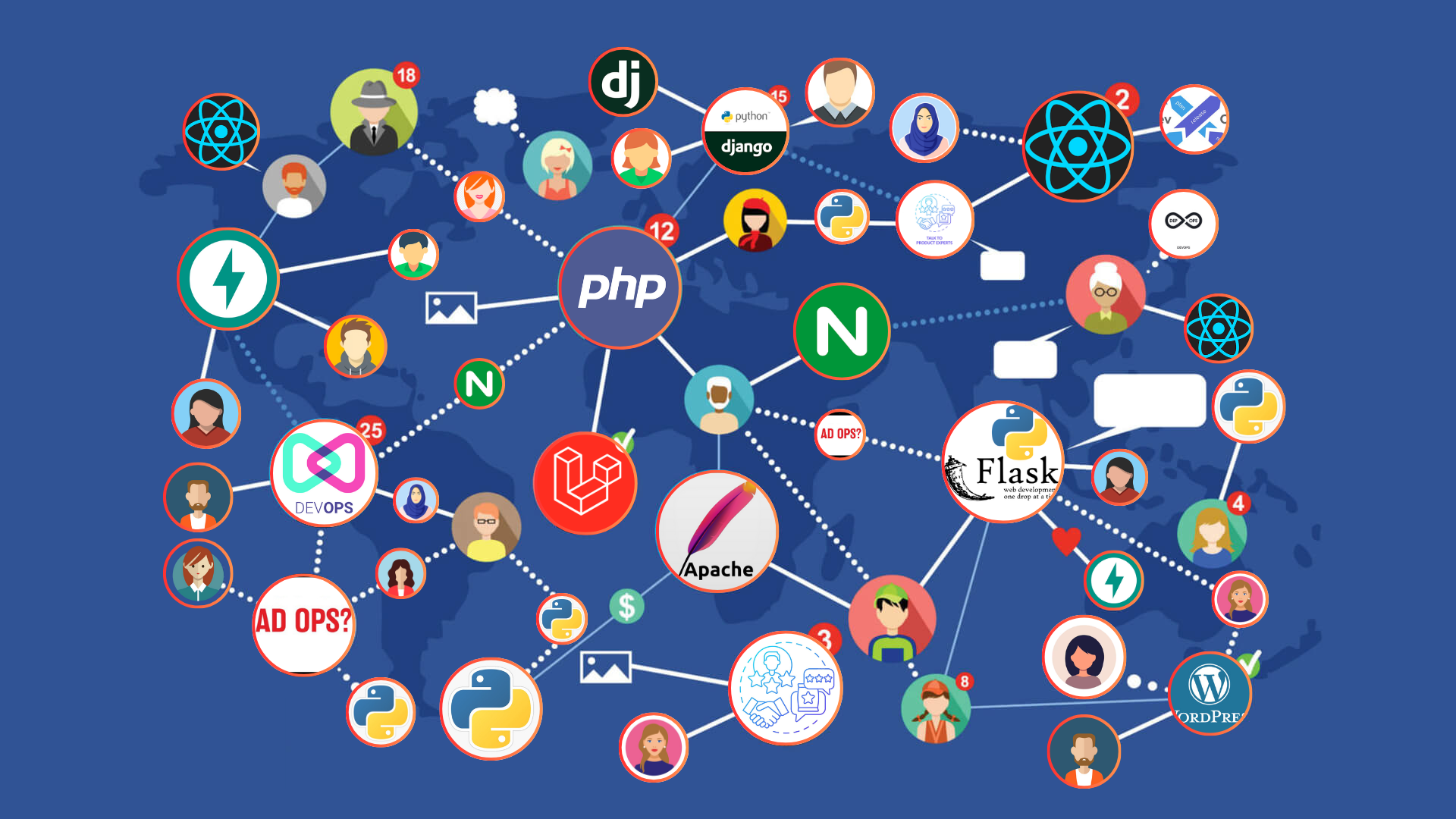
There is a way python native to connect Django to a database through an ssh tunnel. You need to install a package named sshtunnel
.
Requirements: The sshtunnel
package https://github.com/pahaz/sshtunnel
Installation:
sshtunnel is on PyPI, so simply run:
pip install sshtunnel
or
easy_install sshtunnel
or
conda install -c conda-forge sshtunnel
to have it installed in your environment.
For installing from source, clone the repo and run:
python setup.py install
Installation:
In the django settings.py
create an ssh tunnel before the django DB settings block:
from sshtunnel import SSHTunnelForwarder
# Connect to a server using the ssh keys. See the sshtunnel documentation for using password authentication
ssh_tunnel = SSHTunnelForwarder(
SERVER_IP,
ssh_private_key=PATH_TO_SSH_PRIVATE_KEY,
ssh_private_key_password=SSH_PRIVATE_KEY_PASSWORD,
ssh_username=SSH_USERNAME,
remote_bind_address=('localhost', LOCAL_DB_PORT_ON_THE_SERVER),
)
ssh_tunnel.start()
Then add the DB info block in the settings.py
. Here I am adding a default local DB and the remote DB that we connect to using the ssh tunnel
DATABASES = {
'default': {
'ENGINE': 'django.contrib.gis.db.backends.postgis',
'HOST': NORMAL_DB_HOST,
'PORT': NORMAL_DB_PORT,
'NAME': NORMAL_DB_NAME,
'USER': NORMAL_DB_USER,
'PASSWORD': NORMAL_DB_PASSWORD,
},
'shhtunnel_db': {
'ENGINE': 'django.contrib.gis.db.backends.postgis',
'HOST': 'localhost',
'PORT': ssh_tunnel.local_bind_port,
'NAME': REMOTE_DB_DB_NAME,
'USER': REMOTE_DB_USERNAME,
'PASSWORD': REMOTE_DB_PASSWORD,
},
}
That is it. Now one can make migratations to the remote db using commands like $ python manage.py migrate --database=shhtunnel_db
or make calls to the db from within the python code using lines like Models.objects.all().using('shhtunnel_db')
In some case the remote db was created by someone else and You only need to read it. In order to avoid writing the models and deactivating the model manager you can used the following django command to get the models from the database
python manage.py inspectdb
You will see all tables django model in terminal, copy and past it in your expected apps.