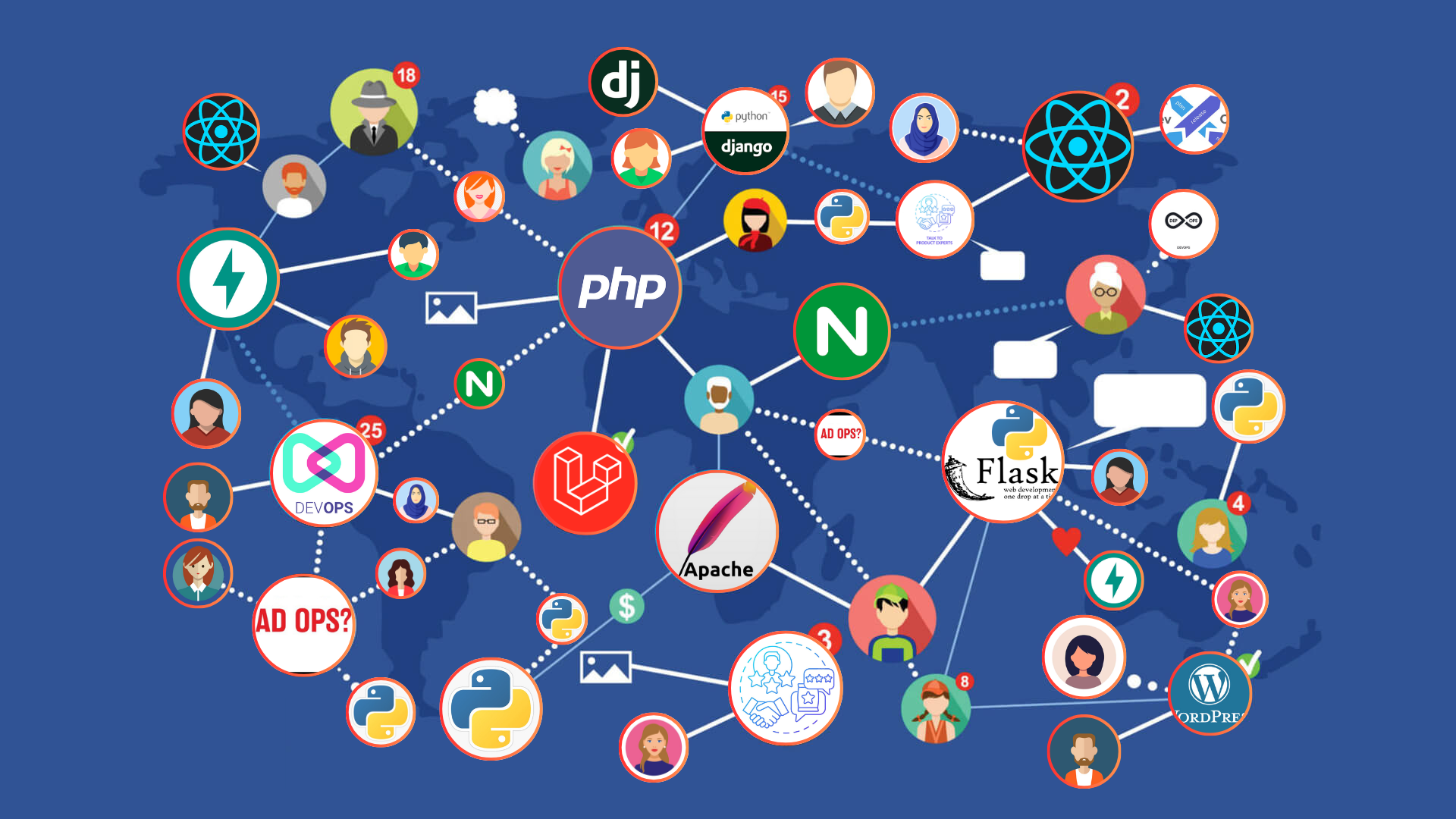
To call a PostgreSQL function from Django, you can use Django's database connection along with the raw
method or the connection.cursor()
method. Here's a basic example using connection.cursor()
:
from django.db import connection
def call_postgresql_function():
with connection.cursor() as cursor:
cursor.callproc('function_name', [param1, param2]) # Replace with your function and parameters
result = cursor.fetchall() # Fetch results if your function returns any
return result
Make sure your PostgreSQL function is created and accessible in the database that your Django application is connected to. Double-check that you have the correct permissions and function parameters.