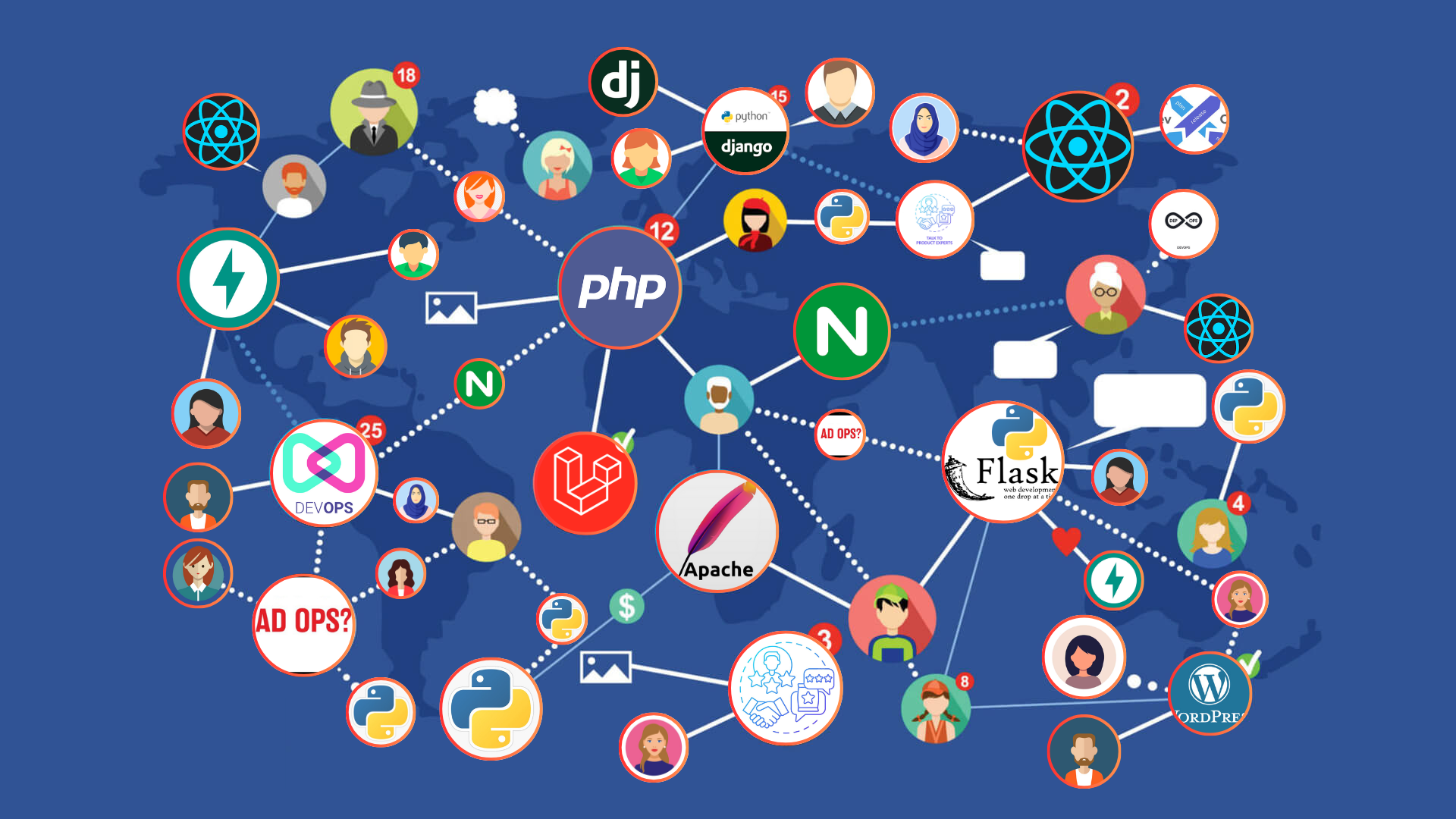
Use operator.itemgetter()
to get multiple items from an iterable in #Python.
The functions fall into categories that perform object comparisons, logical operations, mathematical operations and sequence operations.
The operator
module exports a set of efficient functions corresponding to the intrinsic operators of Python. For example, operator.add(x, y)
is equivalent to the expression x+y
. Many function names are those used for special methods, without the double underscores. For backward compatibility, many of these have a variant with the double underscores kept. The variants without the double underscores are preferred for clarity.
The object comparison functions are useful for all objects, and are named after the rich comparison operators they support:
operator.lt(a, b)
operator.le(a, b)
operator.eq(a, b)
operator.ne(a, b)
operator.ge(a, b)
operator.gt(a, b)
operator.__lt__(a, b)
operator.__le__(a, b)
operator.__eq__(a, b)
operator.__ne__(a, b)
operator.__ge__(a, b)
operator.__gt__(a, b)
Here is a simple example of using this operator function -
In [1]: from operator import itemgetter
In [2]: days=['mon', 'tue', 'wed', 'thurs', 'fri', 'sat', 'sun']
In [3]: days
Out[3]: ['mon', 'tue', 'wed', 'thurs', 'fri', 'sat', 'sun']
In [4]: f = itemgetter(5, 6)
In [5]: f(days)
Out[5]: ('sat', 'sun')
In [6]: workouts={'mon': 'chest+biceps', 'tue': 'legs', 'wed': 'cardio', 'thurs': 'back+triceps', 'fri': 'legs', 'sat':'rest', 'sun': 'rest'}
In [7]: workouts
Out[7]:
{'mon': 'chest+biceps',
'tue': 'legs',
'wed': 'cardio',
'thurs': 'back+triceps',
'fri': 'legs',
'sat': 'rest',
'sun': 'rest'}
In [8]: itemgetter('mon', 'tue')(workouts)
Out[8]: ('chest+biceps', 'legs')
Mapping Operators to Functions
This table shows how abstract operations correspond to operator symbols in the Python syntax and the functions in the operator
module.
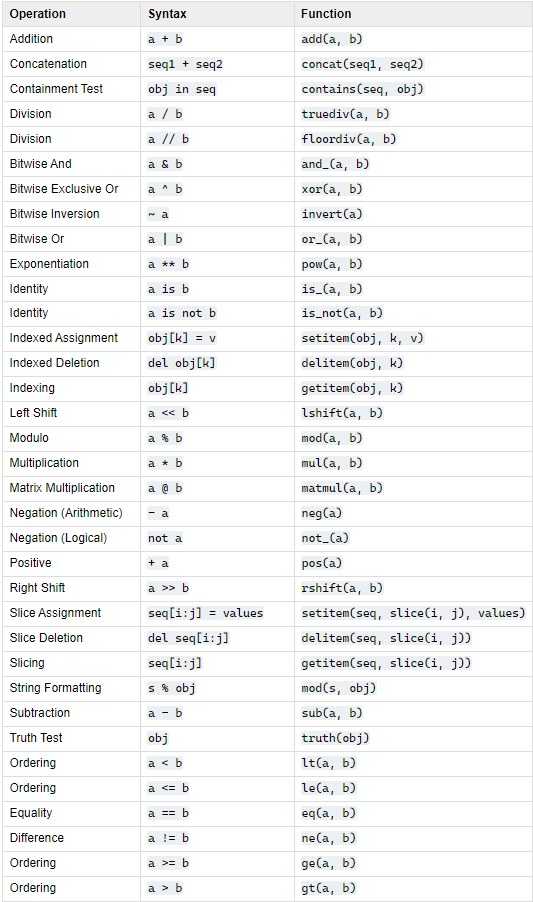